Javascript Variables
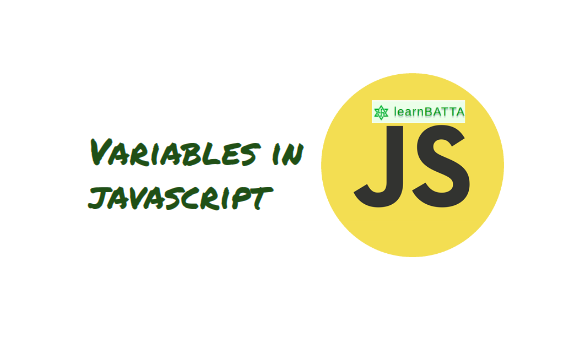
Variables in javascript
- A variable is a name which holds a value in the computer memory.
- Javascript is a dynamic typed language. It means we do not define the types while defning a varialbe unlike the statically typed languages.
- We can store different data types in the variable
Examples for javascript variables
Number variables
let ten = 10;
let weight = 55.5;
String variables
let firstName = "Anjaneyulu";
let lastName = "Batta";
Object variables
let student = {
firstName: "Anjaneyulu",
lastName: "Batta",
age: 20,
weight: 68,
}
let numbers = [1, 2, 3, 4, 5.5]
Points to remember when you are defining a javascript variable
- Variable names cannot contain spaces.
- Variable names must begin with a letter, an underscore (_) or a dollar sign ($).
- Variable names can only contain letters, numbers, underscores, or dollar signs.
- Variable names are case-sensitive(i.e uppercase letters and lowercase letters are not cosidered as same).
- Do not use in-build javascript variables or reserved words.
- Variable should be meaningful & it should represent the information about data stored in it.
- variable name should not be too small or too lengthy
- When you have multiple words in the variable then you can use the camelcase notation or underscore(_) notation.
- Examples:
firstName
, full_address
, collegeName
, address1
, address2
Javascript Reserved Keywords
- JavaScript has some reserved words you should know before you begin coding. The following table contains a list of JavaScript reserved words, which cannot be used as JavaScript variables, functions, methods, loop labels, or object names.
| | | | | |
abstract | boolean | break | byte | case | catch |
char | class | const | continue | debugger | default |
delete | do | double | else | enum | export |
extends | false | final | finally | float | for |
function | goto | if | implements | import | in |
instanceof | int | interface | long | native | new |
null | package | private | protected | public | return |
short | static | super | switch | synchronized | this |
throw | throws | transient | true | try | typeof |
var | void | volatile | while | with | |