Javascript Operators
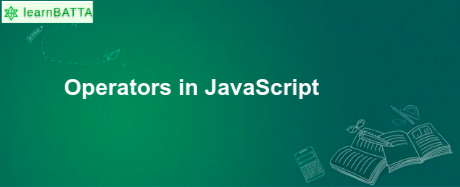
Operators in javascript
JavaScript operators are used to assign values, compare values, perform arithmetic operations, and more.
Assignment (=) Operators
Operator | Description | Example |
= | Assign | 10+10 = 20 |
+= | Add and assign | var a=10; a+=20; Now a = 30 |
-= | Subtract and assign | var a=20; a-=10; Now a = 10 |
*= | Multiply and assign | var a=10; a*=20; Now a = 200 |
/= | Divide and assign | var a=10; a/=2; Now a = 5 |
%= | Modulus and assign | var a=10; a%=2; Now a = 0 |
Arithmetic Operators
Operator | Description | Example |
+ | Addition | 10+20 = 30 |
- | Subtraction | 20-10 = 10 |
* | Multiplication | 10*20 = 200 |
/ | Division | 20/10 = 2 |
% | Modulus (Remainder) | 20%10 = 0 |
++ | Increment | var a=10; a++; Now a = 11 |
-- | Decrement | var a=10; a--; Now a = 9 |
Comparison Operators
Operator | Description | Example |
== | Is equal to | 10==20 = false |
=== | Identical (equal and of same type) | 10==20 = false |
!= | Not equal to | 10!=20 = true |
!== | Not Identical | 20!==20 = false |
> | Greater than | 20>10 = true |
>= | Greater than or equal to | 20>=10 = true |
< | Less than | 20<10 = false |
<= | Less than or equal to | 20<=10 = false |
Logical Operators
Operator | Description | Example |
&& | Logical AND | (10==20 && 20==33) = false |
|| | Logical OR | (10==20 || 20==33) = false |
! | Logical Not | !(10==20) = true |
Ternary Operator
It's the simplest form of writing the if else condition.
Example:
let marks = 30;
let result = marks > 50 ? 'pass' : 'fail';
console.log('result = ', result)