Javascript Class Inheritance
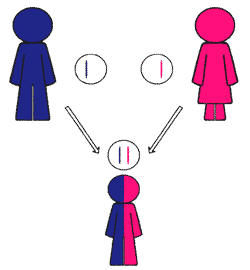
Class inheritance in Javascript
- Inheritance is the procedure in which one class inherits the attributes and methods of another class.
- It provides code reusability
- It gives better maintainability of the code
- Javascript uses
extends
keyword to inherit the class. - Javascript uses
super
keyword to call the parent class method.
A quick example
- Let's write some code to represent the different types cars i.e Audi and Tesla.
- As, we know Audi and Tesla both has common properties and functionalities.
- so, we can have a base class with common properties and functionalities. Let's name it as Car
- The other two classes
Audi
and Tesla
can inherit these properties and can add additional implementations.
class Car {
constructor(color, model, makeYear, fuelType){
this.color = color;
this.model = model;
this.makeYear = makeYear;
this.fuelType = fuelType;
}
start(){
return `started`;
}
stop(){
return `stopped`
}
}
class Audi extends Car{
constructor(color, model, makeYear){
super(color, model, makeYear, 'Petrol')
}
}
class Tesla extends Car{
constructor(color, model, makeYear){
super(color, model, makeYear, 'Electric')
this.autoPilotMode = false;
}
setAutoPilotMode(mode){
this.autoPilotMode = mode;
return this.autoPilotMode;
}
startAutoPilotMode(){
return this.setAutoPilotMode(true);
}
stopAutoPilotMode(){
return this.setAutoPilotMode(false);
}
}
- from the above code, we can see that classes
Audi
, Tesla
both inherited the prperties from class Car
. - We have implemented extra funcionaliy "auto pilot mode" on class
Tesla
. - Let's create objects for both cars
Audi
and Tesla
// create audi object
let audi_a4 = new Audi('Blue', 'A4', 2022);
console.log(audi_a4.start())
// output: started
console.log(audi_a4.color)
// output: Blue
// create tesla object
let tesla = new Tesla('Red', 'S', 2022);
console.log(tesla.start())
// output: started
console.log(tesla.startAutoPilotMode())
// output: true
- Like above code we can re-use the properties from parent class and implement additional properties/functionalities.