Javascript Getters and Setters
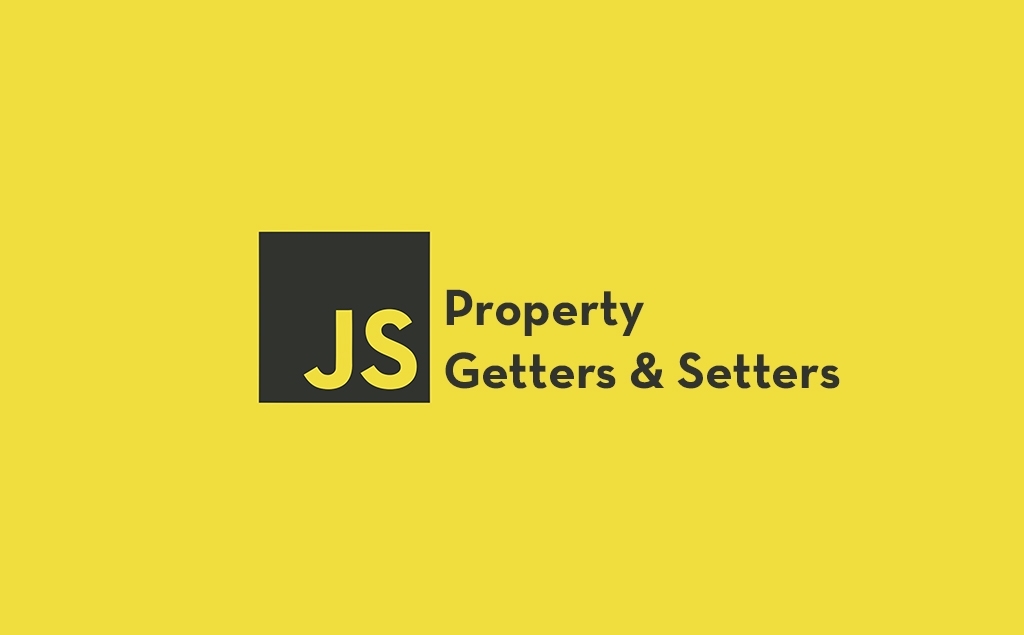
Why to use Getters and Setters?
- We generally define and use the properties in the javascript.
- We access the attribute and change it's value if requied.
- But, some times we may need to return data based on some computations, for that we can use the functions.
- But, can't use it as an attribute but with getters we can access the value and with setters we can set the value.
get
keyword is used for getter and set
keyword is used for setter.
Example use of Getters and Setters
var person = {
firstName: 'Jimmy',
lastName: 'Smith',
get fullName() {
return this.firstName + ' ' + this.lastName;
}
set fullName (name) {
var words = name.toString().split(' ');
this.firstName = words[0] || '';
this.lastName = words[1] || '';
}
}
- we can use
fullName
attribute to get the full name like console.log(person.fullName)
- we can update the
firstName
and lastName
by setting fullName
like below.
person.fullName = 'Vin Diesel'
console.log(person.firstName); // Vin
console.log(person.lastName) // Diesel
Reference: